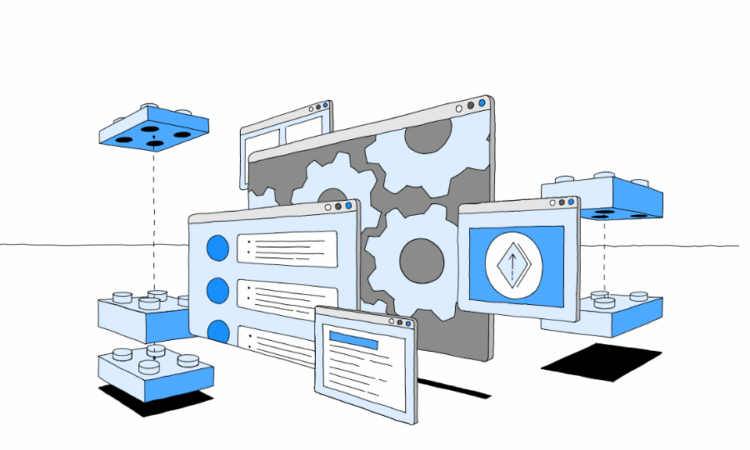
GraphQL has become a preferred choice for building modern full stack applications. It allows developers to fetch only the required data, improving performance and flexibility. However, as applications grow, testing GraphQL resolvers becomes essential to ensure that data fetching and processing work correctly.
Unit testing GraphQL resolvers helps developers catch errors early, improve application stability, and ensure smooth functionality. For those learning web development through a developer course, understanding how to test GraphQL resolvers is a valuable skill.
If you are looking to master full stack development, enrolling in a full stack developer course in Hyderabad can provide hands-on experience in GraphQL and testing strategies. In this blog, we will explore how to perform unit testing for GraphQL resolvers in full stack applications.
What is GraphQL?
It is a query language for APIs that permits clients to request exactly the data they need. Unlike REST APIs, which return fixed structures, GraphQL enables flexible data fetching, reducing over-fetching and under-fetching.
Key Features of GraphQL
- Declarative Data Fetching – Clients specify the required fields, reducing unnecessary data transfer.
- Single Endpoint – Unlike REST, which has multiple endpoints, GraphQL uses a single endpoint for all questions.
- Strongly Typed Schema – The GraphQL schema defines types and relationships, ensuring consistency.
- Efficient Data Loading – GraphQL allows fetching related data in a single request.
For developers taking a Java full stack developer course, learning GraphQL helps in building scalable and efficient APIs.
What are GraphQL Resolvers?
Resolvers are functions that handle GraphQL queries and return data. When a client requests data using a GraphQL query, resolvers execute the logic to retrieve and return the required data.
Example of a GraphQL Resolver (Node.js + Express + Apollo Server)
javascript
CopyEdit
const resolvers = {
Query: {
user: async (_, { id }, { dataSources }) => {
return dataSources.userAPI.getUserById(id);
}
}
};
- The user resolver fetches user data based on an id parameter.
- It retrieves data from dataSources.userAPI.
If a resolver has errors, the GraphQL API will return incorrect or missing data. This is why unit testing resolvers is crucial.
For students enrolled in a full stack developer course in Hyderabad, learning how to write and test GraphQL resolvers can improve application reliability.
Why Unit Testing GraphQL Resolvers is Important
Unit testing ensures that individual resolvers work correctly before integrating them into a full application.
Benefits of Unit Testing GraphQL Resolvers
- Detects Errors Early – Prevents issues before they reach production.
- Ensures Data Accuracy – Confirms that resolvers return the correct data.
- Improves Code Maintainability – Makes it easier to update and refactor resolvers.
- Boosts Developer Confidence – Helps developers trust that their code works correctly.
For those taking a Java full stack developer course, learning unit testing will help them write reliable code that performs well in production.
Setting Up Unit Testing for GraphQL Resolvers
To test GraphQL resolvers, we need a testing framework like Jest and a mock database to simulate API responses.
Step 1: Install Required Dependencies
For a Node.js-based GraphQL server, install the following dependencies:
npm install –save-dev jest @graphql-tools/mock @graphql-tools/schema
- Jest – A JavaScript testing framework.
- @graphql-tools/mock – Allows mocking GraphQL resolvers for testing.
- @graphql-tools/schema – Helps in creating and testing GraphQL schemas.
Step 2: Define the GraphQL Schema
We define a schema to structure our data:
const { makeExecutableSchema } = require(‘@graphql-tools/schema’);
const typeDefs = `
type User {
id: ID!
name: String!
email: String!
}
type Query {
user(id: ID!): User
}
`;
const schema = makeExecutableSchema({ typeDefs });
module.exports = schema;
- This schema defines a User type & a user query to fetch user details.
Writing Unit Tests for GraphQL Resolvers
We will write tests using Jest to verify that resolvers return correct data.
Step 3: Create a Mock Resolver
Mocking allows us to test the resolver without depending on a database.
const resolvers = {
Query: {
user: async (_, { id }) => {
const users = [
{ id: “1”, name: “Alice”, email: “[email protected]” },
{ id: “2”, name: “Bob”, email: “[email protected]” }
];
return users.find(user => user.id === id);
}
}
};
module.exports = resolvers;
- The user resolver fetches a user from a mock dataset instead of a real database.
Step 4: Write Unit Tests
Now, we create tests to check if the resolver works correctly.
const { execute, parse } = require(‘graphql’);
const schema = require(‘./schema’);
const resolvers = require(‘./resolvers’);
describe(‘GraphQL Resolvers’, () => {
test(‘Fetch user by ID’, async () => {
const query = parse(`
query {
user(id: “1”) {
name
}
}
`);
const response = await execute({
schema,
document: query,
rootValue: resolvers,
});
expect(response.data.user.name).toBe(“Alice”);
expect(response.data.user.email).toBe(“[email protected]”);
});
test(‘Returns null for invalid user ID’, async () => {
const query = parse(`
query {
user(id: “99”) {
name
}
}
`);
const response = await execute({
schema,
document: query,
rootValue: resolvers,
});
expect(response.data.user).toBeNull();
});
});
- The first test checks if the resolver correctly returns user details.
- The second test verifies that the resolver returns null for an invalid user ID.
For developers in a Java full stack developer course, writing such tests ensures that GraphQL APIs function as expected.
Best Practices for Testing GraphQL Resolvers
To maintain high-quality tests, follow these best practices:
- Mock Data Instead of Using a Real Database – This makes tests faster and more reliable.
- Test Edge Cases – Ensure tests handle cases like invalid inputs and empty responses.
- Use Jest for Snapshot Testing – Snapshot tests help track changes in response structures.
- Run Tests Before Every Deployment – Automate testing in a CI/CD pipeline for better stability.
For students taking a full stack developer course in Hyderabad, these best practices will help them develop better GraphQL APIs.
Conclusion
Unit testing GraphQL resolvers is essential for ensuring the reliability and accuracy of full stack applications. By writing automated tests, developers can catch bugs early, improve performance, and deliver high-quality applications.
For those looking to advance their web development skills, enlisting in a Java full stack developer course will provide hands-on experience in GraphQL and testing strategies.
If you want to build modern web applications efficiently, consider joining a full stack developer course. With appropriate training, you will gain the expertise needed to write and test GraphQL resolvers effectively, making your applications more robust and scalable.
By mastering GraphQL unit testing, developers can ensure their applications run smoothly and provide users with the best experience possible.
Contact Us:
Name: ExcelR – Full Stack Developer Course in Hyderabad
Address: Unispace Building, 4th-floor Plot No.47 48,49, 2, Street Number 1, Patrika Nagar, Madhapur, Hyderabad, Telangana 500081
Phone: 087924 83183